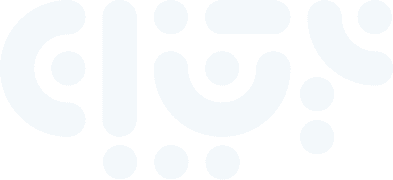
Data Sync - Showcase
This endpoint is currently in beta. It is not yet available in our production URL. Please contact us if you want to use this endpoint. Contact us via hi[at]glair.ai or via our representative for your company.
Showcase Object
- Name
id
- Type
- string
- Description
String representing showcase id.
- Name
name
- Type
- string
- Description
String representing showcase name.
- Name
planogram
- Type
- object | null
- Description
Object container for holding the corresponding planogram to this showcase.
- Name
id
- Type
- string
- Description
The ID of the planogram.
- Name
name
- Type
- string
- Description
String specifying the given name of the planogram.
- Name
products
- Type
- array of Placement
- Description
Array of object container for holding the corresponding placements to this planogram.
- Name
must_have_sku
- Type
- array of SKU
- Description
Array of object container for holding the corresponding must have SKU to this showcase.
- Name
class_id
- Type
- string
- Description
The class ID of the SKU.
- Name
label
- Type
- string
- Description
String specifying the given name of the SKU.
Placement
- Name
row
- Type
- number
- Description
The row where the product should be.
- Name
column
- Type
- string
- Description
The column where the product should be.
- Name
sku
- Type
- object
- Description
Object container for holding the corresponding SKU to this specific placement.
- Name
class_id
- Type
- string
- Description
The class ID of the SKU.
- Name
label
- Type
- string
- Description
String specifying the given name of the SKU.
Get All Showcase
Get list of available showcase.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/showcase';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const config = {
method: 'GET',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
}
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
{
"status": "SUCCESS",
"reason": "Showing all showcase",
"data": [
{
"id": "1",
"name": "Instant Noodle Cup Rack 1",
"planogram": null,
"must_have_sku": [
{
"class_id": 333,
"label": "Mie Sedaap Cup Baso Spesial"
},
{
"class_id": 334,
"label": "Mie Sedaap Cup Goreng"
},
{
"class_id": 335,
"label": "Mie Sedaap Cup Kari Spesial"
},
{
"class_id": 336,
"label": "Mie Sedaap Cup Korean"
}
]
},
{
"id": "2",
"name": "Instant Noodle Cup Rack 2",
"planogram": {
"id": "3",
"name": "Planogram Mie Sedaap",
"products": [
{
"row": 4,
"column": 0,
"sku": {
"class_id": 339,
"label": "Mie Sedaap Instant"
}
},
{
"row": 3,
"column": 0,
"sku": {
"class_id": 336,
"label": "Mie Sedaap Cup Korean"
}
},
{
"row": 2,
"column": 0,
"sku": {
"class_id": 337,
"label": "Mie Sedaap Cup Rawit Bingit"
}
},
{
"row": 1,
"column": 0,
"sku": {
"class_id": 335,
"label": "Mie Sedaap Cup Kari Spesial"
}
},
{
"row": 0,
"column": 0,
"sku": {
"class_id": 333,
"label": "Mie Sedaap Cup Baso Spesial"
}
}
]
},
"must_have_sku": [
{
"class_id": 333,
"label": "Mie Sedaap Cup Baso Spesial"
},
{
"class_id": 335,
"label": "Mie Sedaap Cup Kari Spesial"
},
{
"class_id": 337,
"label": "Mie Sedaap Cup Rawit Bingit"
}
]
}
]
}
Get Showcase by Id
Get showcase by identifier.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/showcase/1';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const config = {
method: 'GET',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
}
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
{
"status": "SUCCESS",
"reason": "Showing showcase by identifier: 1",
"data": {
"id": "1",
"name": "Instant Noodle Cup Rack 1",
"planogram": null,
"must_have_sku": [
{
"class_id": 333,
"label": "Mie Sedaap Cup Baso Spesial"
},
{
"class_id": 334,
"label": "Mie Sedaap Cup Goreng"
},
{
"class_id": 335,
"label": "Mie Sedaap Cup Kari Spesial"
},
{
"class_id": 336,
"label": "Mie Sedaap Cup Korean"
}
]
}
}
Create Showcase
Create showcase.
Required Request Payload
- Name
id
- Type
- string
- Description
String representing showcase id.
- Name
name
- Type
- string
- Description
String representing showcase name.
Optional Request Payload
- Name
planogram_id
- Type
- string
- Description
String representing If of the planogram.
- Name
must_have_sku
- Type
- array of string
- Description
Array of sku id container for holding the corresponding must have SKU to this showcase.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/showcase';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const requestBody = {
id: '3',
name: 'Instant Noodle Cup Rack 3',
planogram_id: '2',
must_have_sku: ['333']
};
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
'Content-Type': 'application/json'
},
body: JSON.stringify(requestBody)
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "Showcase successfully created"
}
Update Showcase
Update showcase by identifier.
Optional Request Payload
- Name
name
- Type
- string
- Description
String representing showcase name.
- Name
planogram_id
- Type
- string
- Description
String representing If of the planogram.
- Name
must_have_sku
- Type
- array of string
- Description
Array of sku id container for holding the corresponding must have SKU to this showcase.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/showcase/3';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const requestBody = {
name: 'Instant Noodle Cup Rack 3',
must_have_sku: ["334"]
};
const config = {
method: 'PUT',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
'Content-Type': 'application/json'
},
body: JSON.stringify(requestBody)
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "Showcase successfully updated by identifier: 3"
}
Delete Showcase
Delete showcase by identifier.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/showcase/3';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const config = {
method: 'DELETE',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey
}
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "Showcase successfully deleted by identifier: 3"
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with valid data
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "Showing all showcase",
//...,
}
400 - Bad Request
Request with invalid data
Response
1
2
3
4
{
"status": "FAILED",
"reason": "Showcase id does not exist",
}
500 - Internal Server Error
Failed request
Response
1
2
3
4
{
"status": "FAILED",
"reason": "Internal server error",
}