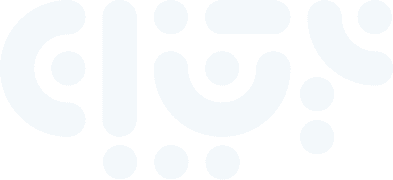
Quickstart
We'll cover how to get started using one of our SDKs and how to make your first API request.
Before you can make requests to the GLAIR Vision API, you must obtain API Key, username, and password. For now, you can obtain them by contacting us via hi[at]glair.ai or via our representative for your company. In the future, we will release a dashboard to make it easier for you to manage your credentials.
Choose your SDK
Before making your first API request, you need to pick which SDK you will use. In the following section, we provide code samples for NodeJS, Java, Go, and cURL.
1
2
3
# Install the GLAIR Vision NodeJS SDK
# Requires Node.js v18 or higher.
npm install --save @glair/vision
Making your first API request
After picking your preferred SDK, you are ready to make your first call to the GLAIR Vision API. Below is the example of calling the KTP endpoint with our SDKs.
1
2
3
4
5
6
7
8
9
import { Vision } from '@glair/vision';
const vision = new Vision({
apiKey: 'api-key',
username: 'username',
password: 'password',
});
await vision.ocr.ktp('/path/to/image/KTP.jpg');
Making request without SDK
If you prefer to not use our SDKs, you still can do it by yourself. Just with a little more boilerplate. See Authentication for more details on authenticating your request.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
// Install the required dependencies: 'npm install --save node-fetch form-data-encoder formdata-node'
// Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import fetch from 'node-fetch';
import { FormDataEncoder } from 'form-data-encoder';
import { FormData, Blob } from 'formdata-node';
import { fileFromPath } from 'formdata-node/file-from-path';
const url = 'https://api.vision.glair.ai/ocr/v1/ktp';
const basicAuth = 'Basic ' + btoa('USERNAME' + ':' + 'PASSWORD');
const apiKey = 'API_KEY';
const data = new FormData();
data.append('image', await fileFromPath('/path/to/image/KTP.jpeg'));
const encoder = new FormDataEncoder(data);
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
},
body: new Blob(encoder, { type: encoder.contentType }),
};
const response = await fetch(url, config);
console.log(await response.json());
What's next?
Great, you're now set up with an SDK and have made your first request to the API. Here are a few links that might be handy as you venture further into the GLAIR Vision API: