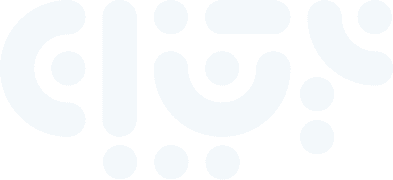
POSM Analysis
POSM is a metric that measures the effectiveness and impact of point-of-sale materials and promotional activities in driving sales and influencing consumer behavior.
This endpoint is currently in beta. It is not yet available in our production URL. Please contact us if you want to use this endpoint. Contact us via hi[at]glair.ai or via our representative for your company.
POSM Analysis Object
- Name
status
- Type
- string
- Description
Enum code indicating the status of the reading result.
SUCCESS
NO_FILE
FILE_INVALID_FORMAT
FAILED
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
posm_score
- Type
- number
- Description
Integer representing POSM availability compliance score in percentage.
- Name
detected_object
- Type
- array of DetectedObject
- Description
Array of object containing detected POSM provided by object detection AI service.
- Name
item
- Type
- object
- Description
Object container for holding all the information related to the detected item.
- Name
class_id
- Type
- string
- Description
The class ID of the detected object.
- Name
label
- Type
- string
- Description
String specifying the given name of the detected object/product.
- Name
coordinates
- Type
- object
- Description
The exact pixel coordinate that represents the rectangular area in the image where the object is detected. - [xmin, ymin]: upper-left corner coordinate of the object. - [xmax, ymax]: lower-right corner coordinate of the object.
- Name
confidence
- Type
- number
- Description
Float, score representing the likelihood that the output given by the AI service/model is correct, the higher the score means better result.
Analyze POSM KPI
Calculate POSM score using the response received from computer vision.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg)
- Description
The image file.
- Name
showcaseId
- Type
- string
- Description
The showcase id.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/kpi/posm';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const data = new FormData();
data.append('image', new Blob(
[readFileSync('/path/to/image/image.jpg')],
{ type: 'image/jpg' }
));
data.append('showcaseId', '1');
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
},
body: data,
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
{
"status": "SUCCESS",
"reason": "File successfully read",
"posm_score": 100,
"detected_object": [
{
"class_id": 5,
"coordinate": {
"xmin": 6,
"ymin": 911,
"xmax": 268,
"ymax": 1164
},
"label": "wakuwaku-tebak-rasa",
"confidence": 0.9975013136863708
},
{
"class_id": 0,
"coordinate": {
"xmin": 1388,
"ymin": 927,
"xmax": 1628,
"ymax": 1163
},
"label": "frostbite-chocolate-strawberry",
"confidence": 0.9959197044372559
}
]
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with readable image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File successfully read",
//...,
}
400 - Bad Request
Request without form-data image
Response
1
2
3
4
{
"status": "NO_FILE",
"reason": "No file in request body",
}
415 - Unsupported Media Type
Request with non-image file format
Response
1
2
3
4
{
"status": "FILE_INVALID_FORMAT",
"reason": "Failed to process invalid request",
}