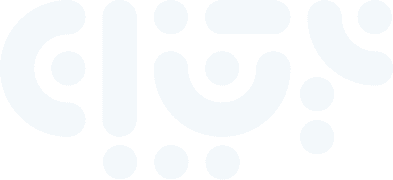
KPI - Share of Shelf Analysis
Share of shelf is a metric that measures the proportion or percentage of shelf space occupied by a particular brand compared to all brands shown in the same shelf.
This endpoint is currently in beta. It is not yet available in our production URL. Please contact us if you want to use this endpoint. Contact us via hi[at]glair.ai or via our representative for your company.
Share of Shelf Analysis Object
- Name
status
- Type
- string
- Description
Enum code indicating the status of the reading result.
SUCCESS
NO_FILE
FILE_INVALID_FORMAT
FAILED
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
share_of_shelf
- Type
- object
- Description
Object containing Share of Shelf result calculated using the response by object detection AI service.
- Name
own_product_score
- Type
- number
- Description
Integer representing proportion of own product's score in percentage.
- Name
competitor_product_score
- Type
- number
- Description
Integer representing proportion of other or competitor's product score in percentage.
- Name
competitor_products_detail
- Type
- array of CompetitorShare
- Description
Array of proportion of other/competitor's product and score.
- Name
brand
- Type
- string
- Description
Other / competitor's brand name.
- Name
score
- Type
- number
- Description
Integer representing proportion of other or competitor's product score in percentage.
Analyze Share of Shelf KPI
Calculate Share of Shelf score using the response received from computer vision.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg)
- Description
The image file.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/kpi/sos';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const data = new FormData();
data.append('image', new Blob(
[readFileSync('/path/to/image/image.jpg')],
{ type: 'image/jpg' }
));
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
},
body: data,
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
{
"status": "SUCCESS",
"reason": "File successfully read",
"share_of_shelf": {
"own_product_score": 77.78,
"competitor_product_score": 22.22,
"competitor_products": [
{
"brand": "Pop Mie",
"score": 11.11
},
{
"brand": "Gaga",
"score": 11.11
}
]
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with readable image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File successfully read",
//...,
}
400 - Bad Request
Request without form-data image
Response
1
2
3
4
{
"status": "NO_FILE",
"reason": "No file in request body",
}
415 - Unsupported Media Type
Request with non-image file format
Response
1
2
3
4
{
"status": "FILE_INVALID_FORMAT",
"reason": "Failed to process invalid request",
}