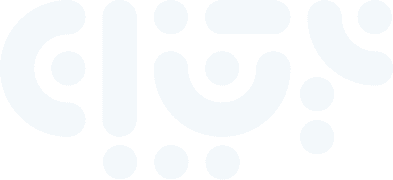
Data Sync - SKU
This endpoint is currently in beta. It is not yet available in our production URL. Please contact us if you want to use this endpoint. Contact us via hi[at]glair.ai or via our representative for your company.
SKU Object
- Name
id
- Type
- string
- Description
String representing sku id.
- Name
label
- Type
- string
- Description
String representing sku label.
- Name
color
- Type
- string
- Description
String representing sku color.
Get All SKU
Get list of available SKU.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/sku';
const apiKey = API_KEY;
const config = {
method: 'GET',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
}
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
{
"status": "SUCCESS",
"reason": "Showing all SKU",
"data": [
{
"id": 333,
"label": "Mie Sedaap Cup Baso Spesial",
"color": "#FF0000"
},
{
"id": 334,
"label": "Mie Sedaap Cup Goreng",
"color": "#FF0000"
},
{
"id": 335,
"label": "Mie Sedaap Cup Kari Spesial",
"color": "#FF0000"
},
{
"id": 336,
"label": "Mie Sedaap Cup Korean",
"color": "#FF0000"
}
]
}
Get SKU by Id
Get SKU by identifier.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/sku/336';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const config = {
method: 'GET',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
}
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
{
"status": "SUCCESS",
"reason": "Showing SKU by identifier: 336",
"data": {
"id": 336,
"label": "Mie Sedaap Cup Korean",
"color": "#FF0000"
}
}
Create SKU
Create SKU.
Required Request Payload
- Name
id
- Type
- string
- Description
String representing SKU id.
- Name
label
- Type
- string
- Description
String representing SKU label.
Optional Request Payload
- Name
color
- Type
- string
- Description
String representing SKU color.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/sku';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const requestBody = {
id: 337,
label: "Mie Sedaap Cup Korean",
color: "#FF0000"
};
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
'Content-Type': 'application/json'
},
body: JSON.stringify(requestBody)
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "SKU successfully created"
}
Update SKU
Update SKU by identifier.
Optional Request Payload
- Name
label
- Type
- string
- Description
String representing SKU label.
- Name
color
- Type
- string
- Description
String representing SKU color.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/sku/337';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const requestBody = {
label: "Mie Sedaap Cup Korean Special",
};
const config = {
method: 'PUT',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
'Content-Type': 'application/json'
},
body: JSON.stringify(requestBody)
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "SKU successfully updated by identifier: 337"
}
Delete SKU
Delete showcase by identifier.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/sku/337';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const config = {
method: 'DELETE',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey
}
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "SKU successfully deleted by identifier: 337"
}
Sync SKU
Synchronize showcase.
Request Payload
- Name
payload
- Type
- array of synchronized SKU
- Description
Array of synchronized SKU request.
Required Synchronized SKU Payload
- Name
active
- Type
- boolean
- Description
Boolean representing is the SKU still active.
Optional Synchronized SKU Payload
- Name
label
- Type
- string
- Description
String representing SKU label.
- Name
color
- Type
- string
- Description
String representing SKU color.
Explanation
- If the current SKU in database is not provided in the payload, it remains unchanged.
- If the current SKU in database is in the payload and the active state in payload is true, it will be updated.
- If the current SKU in database is in the payload and the active state in payload is false, it will be deleted.
- If the SKU in the payload is not found in database, a new SKU will be created.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/data/sku/sync';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const requestBody = {
payload: [
{
id: 334,
label: "Mie Sedaap Cup Goreng",
active: false
},
{
id: 338,
label: "Mie Sedaap Cup Ramen",
active: true
},
{
id: 339,
label: "Mie Sedaap Cup Soto",
active: true
}
]
};
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey
},
body: JSON.stringify(requestBody)
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
{
"status": "SUCCESS",
"reason": "SKU successfully synchronized"
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with valid data
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "Showing all SKU",
//...,
}
400 - Bad Request
Request with invalid data
Response
1
2
3
4
{
"status": "FAILED",
"reason": "SKU id does not exist",
}
500 - Internal Server Error
Failed request
Response
1
2
3
4
{
"status": "FAILED",
"reason": "Internal server error",
}