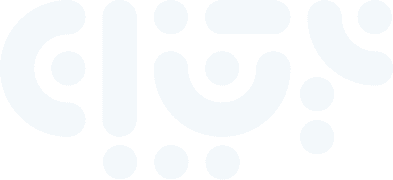
KPI - On Shelf Availability Analysis
On shelf availability is a metric that measures the availability of products on store shelves, indicating the percentage of items that are properly stocked and accessible to customers.
This endpoint is currently in beta. It is not yet available in our production URL. Please contact us if you want to use this endpoint. Contact us via hi[at]glair.ai or via our representative for your company.
On Shelf Availability Analysis Object
- Name
status
- Type
- string
- Description
Enum code indicating the status of the reading result.
SUCCESS
NO_FILE
FILE_INVALID_FORMAT
FAILED
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
on_shelf_availability
- Type
- object
- Description
Object containing On Shelf Availability result calculated using the response by object detection AI service.
- Name
score
- Type
- number
- Description
Integer representing on shelf availability score in percentage.
- Name
sku_detected
- Type
- array of ProductWithIndex
- Description
Array of containing detected SKU that match with the must have reference for On Shelf Availability.
- Name
sku_missing
- Type
- array of Product
- Description
Array of containing must have reference that missing from the list of detected SKU.
- Name
sku_excluded
- Type
- array of ProductWithIndex
- Description
Array of containing detected SKU that not match with the must have reference for On Shelf Availability.
- Name
detected_object
- Type
- array of DetectedObject
- Description
Array of object containing result provided by object detection AI service.
- Name
index
- Type
- number
- Description
Integer for indexing purpose, specifically referred by the items field that can be found in on shelf availability subsection.
- Name
item
- Type
- object
- Description
Object container for holding all the information related to the detected item.
- Name
class_id
- Type
- string
- Description
The class ID of the detected object.
- Name
label
- Type
- string
- Description
String specifying the given name of the detected object/product.
- Name
coordinates
- Type
- object
- Description
The exact pixel coordinate that represents the rectangular area in the image where the object is detected. - [xmin, ymin]: upper-left corner coordinate of the object. - [xmax, ymax]: lower-right corner coordinate of the object.
- Name
confidence
- Type
- number
- Description
Float, score representing the likelihood that the output given by the AI service/model is correct, the higher the score means better result.
- Name
position
- Type
- object
- Description
Object representing relative position of the object on the planogram (based on shelf-partition / row-column metrics).
- Name
shelf
- Type
- string
- Description
The shelf in which the object is located. If there are no shelves in the image, this will be 0 (Start from index 0).
- Name
partition
- Type
- number
- Description
The partition in which the object is located. If there are no partitions, this will be 0 (Start from index 0).
Product
- Name
class_id
- Type
- string
- Description
The class ID of the detected object.
- Name
label
- Type
- string
- Description
String specifying the given name of the detected object/product.
ProductWithIndex
- Name
class_id
- Type
- string
- Description
The class ID of the detected object.
- Name
label
- Type
- string
- Description
String specifying the given name of the detected object/product.
- Name
count
- Type
- number
- Description
Total products.
- Name
indexes
- Type
- array of number
- Description
The list of numbers represents indexes in the detected object array.
Analyze On Shelf Availability KPI
Calculate On Shelf Availability score using the response received from computer vision.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg)
- Description
The image file.
- Name
showcaseId
- Type
- string
- Description
The showcase id.
Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from 'fs';
const url = 'https://api.vision.glair.ai/retail/v1/kpi/osa';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = API_KEY;
const data = new FormData();
data.append('image', new Blob(
[readFileSync('/path/to/image/image.jpg')],
{ type: 'image/jpg' }
));
data.append('showcaseId', '1');
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
},
body: data,
};
const response = await fetch(url, config);
console.log(await response.json());
Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
{
"status": "SUCCESS",
"reason": "File successfully read",
"on_shelf_availability": {
"score": 66.67,
"detected": [
{
"class_id": 338,
"label": "Mie Sedaap Cup Soto",
"count": 3,
"indexes": [0, 1, 4]
},
{
"class_id": 337,
"label": "Mie Sedaap Cup Rawit Bingit 75g",
"count": 2,
"indexes": [5, 6]
}
],
"missing": [
{
"class_id": 336,
"label": "Mie Sedaap Cup Korean"
}
],
"excluded": [
{
"class_id": 335,
"label": "Mie Sedaap Cup Kari Spesial 81g",
"count": 2,
"indexes": [2, 3]
},
{
"class_id": 170,
"label": "Gaga Mi Instan Cup 75g",
"count": 1,
"indexes": [8]
},
{
"class_id": 437,
"label": "Pop Mie Cup",
"count": 1,
"indexes": [7]
},
]
},
"detected_object": [
{
"index": 0,
"item": {
"class_id": 338,
"coordinates": {
"xmin": 6,
"ymin": 120,
"xmax": 96,
"ymax": 238
},
"label": "Mie Sedaap Cup Soto",
"confidence": 0.463654100894928,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 0,
"partition": 0
}
},
{
"index": 1,
"item": {
"class_id": 338,
"coordinates": {
"xmin": 17,
"ymin": 329,
"xmax": 115,
"ymax": 431
},
"label": "Mie Sedaap Cup Soto",
"confidence": 0.4637259542942047,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 0,
"partition": 0
}
},
{
"index": 2,
"item": {
"class_id": 335,
"coordinates": {
"xmin": 23,
"ymin": 489,
"xmax": 107,
"ymax": 585
},
"label": "Mie Sedaap Cup Kari Spesial 81g",
"confidence": 0.5406119227409363,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 1,
"partition": 0
}
},
{
"index": 3,
"item": {
"class_id": 335,
"coordinates": {
"xmin": 24,
"ymin": 582,
"xmax": 110,
"ymax": 683
},
"label": "Mie Sedaap Cup Kari Spesial 81g",
"confidence": 0.5788369178771973,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 1,
"partition": 0
}
},
{
"index": 4,
"item": {
"class_id": 338,
"coordinates": {
"xmin": 24,
"ymin": 226,
"xmax": 112,
"ymax": 331
},
"label": "Mie Sedaap Cup Soto",
"confidence": 0.5329965353012085,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 0,
"partition": 0
}
},
{
"index": 5,
"item": {
"class_id": 337,
"coordinates": {
"xmin": 42,
"ymin": 735,
"xmax": 125,
"ymax": 828
},
"label": "Mie Sedaap Cup Rawit Bingit 75g",
"confidence": 0.5875117778778076,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 2,
"partition": 0
}
},
{
"index": 6,
"item": {
"class_id": 337,
"coordinates": {
"xmin": 43,
"ymin": 819,
"xmax": 132,
"ymax": 913
},
"label": "Mie Sedaap Cup Rawit Bingit 75g",
"confidence": 0.5880478024482727,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Mie Sedaap"
}
]
},
"position": {
"shelf": 2,
"partition": 0
}
},
{
"index": 7,
"item": {
"class_id": 437,
"coordinates": {
"xmin": 109,
"ymin": 487,
"xmax": 201,
"ymax": 583
},
"label": "Pop Mie",
"confidence": 0.4853852689266205,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Pop Mie"
}
]
},
"position": {
"shelf": 1,
"partition": 0
}
},
{
"index": 8,
"item": {
"class_id": 170,
"coordinates": {
"xmin": 127,
"ymin": 735,
"xmax": 215,
"ymax": 831
},
"label": "Gaga Mi Instan Cup 75g",
"confidence": 0.6708394885063171,
"color": "#6A0C4F",
"group": [
{
"label": "category",
"value": "Instant Noodle"
},
{
"label": "brand",
"value": "Gaga"
}
]
},
"position": {
"shelf": 2,
"partition": 0
}
}
]
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with readable image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File successfully read",
//...,
}
400 - Bad Request
Request without form-data image
Response
1
2
3
4
{
"status": "NO_FILE",
"reason": "No file in request body",
}
415 - Unsupported Media Type
Request with non-image file format
Response
1
2
3
4
{
"status": "FILE_INVALID_FORMAT",
"reason": "Failed to process invalid request",
}