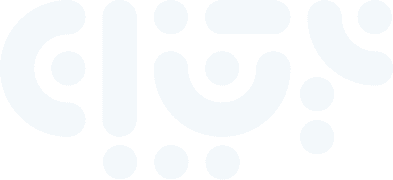
Face Matching
Face matching or verification is the task of comparing a candidate face to another, and verifying whether it is a match or not. It is a one-to-one mapping.
Face Matching Object
- Name
status
- Type
- string
- Description
A short string indicating the status of the result.
success
failed
- Name
result
- Type
- object
- Description
The result of face matching.
- Name
match_percentage
- Type
- number
- Description
How much the faces match each other (in percentage).
- Name
match_status
- Type
- string
- Description
A human-readable message providing more details about the match.
- Name
match_status_code
- Type
- string
- Description
Enum code indicating
match_status
.MATCH
NOT_MATCH
NO_FACE_STORED_IMG
NO_FACE_CAPTURED_IMG
MANY_FACES_STORED_IMG
MANY_FACES_CAPTURED_IMG
Face Match
Match a captured image to the stored one.
Required parameter
- Name
captured_image
- Type
- file (.png, .jpg, .jpeg)
- Description
The captured image needs to be compared with the stored image. It is in Base64 format and does not have an extension prefix.
Unacceptable Base64:
data:image/png;base64,iVBORw0K...
Acceptable Base64:
iVBORw0K...
- Name
stored_image
- Type
- file (.png, .jpg, .jpeg)
- Description
The image you store that is used for ground truth. It is in Base64 format and does not have an extension prefix.
Sample Request
1
2
3
4
5
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.faceBio.match({ captured: '/path/to/image/Captured.jpg', stored: '/path/to/image/Stored.jpg' });
Response
1
2
3
4
5
6
7
8
{
"status": "success",
"result": {
"match_percentage": 0.95768,
"match_status": "match",
"match_status_code": "MATCH"
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Success, matches
Response
1
2
3
4
5
6
7
8
{
"status": "success",
"result": {
"match_percentage": 0.95768,
"match_status": "match",
"match_status_code": "MATCH"
}
}
200 - OK
Success, does not match.
Response
1
2
3
4
5
6
7
8
{
"status": "success",
"result": {
"match_percentage": 0.54321,
"match_status": "not match",
"match_status_code": "NOT_MATCH"
}
}
200 - OK
Failed, too many faces in stored_image
.
Response
1
2
3
4
5
6
7
8
{
"status": "failed",
"result": {
"match_percentage": 0.0,
"match_status": "stored image not detected",
"match_status_code": "NO_FACE_STORED_IMG"
}
}
200 - OK
Failed, no face detected captured_image
.
Response
1
2
3
4
5
6
7
8
{
"status": "failed",
"result": {
"match_percentage": 0.0,
"match_status": "captured image not detected",
"match_status_code": "NO_FACE_CAPTURED_IMG"
}
}
200 - OK
Failed, too many faces in stored_image
.
Response
1
2
3
4
5
6
7
8
{
"status": "failed",
"result": {
"match_percentage": 0.0,
"match_status": "too many faces for stored image",
"match_status_code": "MANY_FACES_STORED_IMG"
}
}
200 - OK
Failed, too many faces in captured_image
.
Response
1
2
3
4
5
6
7
8
{
"status": "failed",
"result": {
"match_percentage": 0.0,
"match_status": "too many faces for captured image",
"match_status_code": "MANY_FACES_CAPTURED_IMG"
}
}
400 - Bad Request
Invalid base64 format for captured_image
.
Response
1
2
3
4
{
"status": "failed",
"error_message": "enter valid base64 for captured image",
}
400 - Bad Request
Invalid base64 format for stored_image
.
Response
1
2
3
4
{
"status": "failed",
"error_message": "enter valid base64 for stored image",
}
400 - Bad Request
Invalid file format for captured_image
.
Response
1
2
3
4
{
"status": "failed",
"error_message": "enter valid format image for captured image",
}
400 - Bad Request
Invalid file format for stored_image
.
Response
1
2
3
4
{
"status": "failed",
"error_message": "enter valid format image for stored image",
}
500 - Internal Server Error
GLAIR Vision API server error.
Response
1
2
3
4
{
"status": "failed",
"error_message": "internal server error"
}