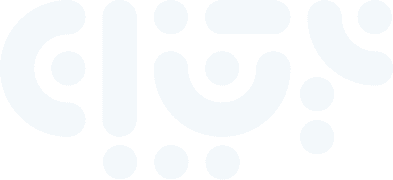
Basic Verification (KTP)
Verify certain KTP fields and personal information using the NIK against the Dukcapil database. It will only return properties with the specified status.
Basic Verification Object
- Name
verification_status
- Type
- boolean
- Description
If
true
, the uploaded KTP data is valid and correct.
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
data
- Type
- object
- Description
Contains the verification status for each inputted KTP fields.
- Name
nik
- Type
- boolean | null
- Description
If
true
, the uploaded NIK is valid and exists in Dukcapil database.
- Name
name
- Type
- boolean | null
- Description
If
true
, the uploaded name field is valid and corresponds with given NIK.
- Name
date_of_birth
- Type
- boolean | null
- Description
If
true
, the uploaded date of birth field is valid and corresponds with given NIK.
- Name
no_kk
- Type
- boolean | null
- Description
If
true
, the uploaded KK number field is valid and corresponds with given information.
- Name
mother_maiden_name
- Type
- boolean | null
- Description
If
true
, the uploaded mother maiden name field is valid and corresponds with given information.
- Name
place_of_birth
- Type
- boolean | null
- Description
If
true
, the uploaded place of birth field is valid and corresponds with given information.
- Name
address
- Type
- boolean | null
- Description
If
true
, the uploaded address field is valid and corresponds with given information.
- Name
gender
- Type
- boolean | null
- Description
If
true
, the uploaded gender field is valid and corresponds with given information.
- Name
marital_status
- Type
- boolean | null
- Description
If
true
, the uploaded marital status field is valid and corresponds with given information.
- Name
job_type
- Type
- boolean | null
- Description
If
true
, the uploaded job type field is valid and corresponds with given information.
- Name
province
- Type
- boolean | null
- Description
If
true
, the uploaded province field is valid and corresponds with given information.
- Name
city
- Type
- boolean | null
- Description
If
true
, the uploaded city field is valid and corresponds with given information.
- Name
district
- Type
- boolean | null
- Description
If
true
, the uploaded district field is valid and corresponds with given information.
- Name
subdistrict
- Type
- boolean | null
- Description
If
true
, the uploaded subdistrict field is valid and corresponds with given information.
- Name
rt
- Type
- boolean | null
- Description
If
true
, the uploaded rt field is valid and corresponds with given information.
- Name
rw
- Type
- boolean | null
- Description
If
true
, the uploaded rw field is valid and corresponds with given information.
Basic Verification
Verify some KTP field by NIK to Dukcapil database and return the verification result.
Required parameter
- Name
nik
- Type
- string
- Description
The
NIK
field in KTP.
Optional parameter
- Name
name
- Type
- string
- Description
The
Nama
field in KTP.
- Name
date_of_birth
- Type
- string
- Description
The
Tanggal Lahir
field in KTP withdd-mm-yyyy
format.
- Name
no_kk
- Type
- string
- Description
The
No Kartu Keluarga
associated with the entered name.
- Name
mother_maiden_name
- Type
- string
- Description
The
Nama Ibu Kandung
associated with the entered name.
- Name
place_of_birth
- Type
- string
- Description
The
Tempat Lahir
field in KTP.
- Name
address
- Type
- string
- Description
The
Alamat
field in KTP.
- Name
gender
- Type
- string
- Description
The
Jenis Kelamin
field in KTP.
- Name
marital_status
- Type
- string
- Description
The
Status Perkawinan
field in KTP.
- Name
job_type
- Type
- string
- Description
The
Pekerjaan
field in KTP.
- Name
province
- Type
- string
- Description
The
Provinsi
field in KTP.
- Name
city
- Type
- string
- Description
The
Kota
field in KTP.
- Name
district
- Type
- string
- Description
The
Kecamatan
field in KTP.
- Name
subdistrict
- Type
- string
- Description
The
Kel/Desa
field in KTP.
- Name
rt
- Type
- string
- Description
The
RT
field in KTP.
- Name
rw
- Type
- string
- Description
The
RW
field in KTP.
Sample Request
1
2
3
4
5
6
7
8
9
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.identity.verification({
nik: 'NIK',
name: 'Nama',
date_of_birth: '01-02-1999', // dd-mm-yyyy
});
Sample Response
1
2
3
4
5
6
7
8
9
{
"verification_status": true,
"reason": "Verification Success",
"result": {
"nik": true,
"name": true,
"date_of_birth": true
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint.
200 - OK
Request with valid and verified KTP data
Response
1
2
3
4
5
6
7
8
9
{
"verification_status": true,
"reason": "Verification Success",
"result": {
"nik": true,
"name": true,
"date_of_birth": true
}
}
200 - OK
Request with a NIK doesn't exist in Dukcapil
Response
1
2
3
4
5
6
7
8
9
{
"verification_status": false,
"reason": "NIK not found on Dukcapil database",
"result": {
"nik": false,
"name": null,
"date_of_birth": null
}
}
200 - OK
Request with field name doesn't match with given NIK
Response
1
2
3
4
5
6
7
8
9
{
"verification_status": false,
"reason": "Name does not match",
"result": {
"nik": true,
"name": false,
"date_of_birth": true
}
}
200 - OK
Request with field date of birth doesn't match with given NIK
Response
1
2
3
4
5
6
7
8
9
{
"verification_status": false,
"reason": "Date of birth does not match",
"result": {
"nik": true,
"name": true,
"date_of_birth": false
}
}
400 - Bad Request
Request with invalid NIK number
Response
1
2
3
4
{
"verification_status": false,
"reason": "Invalid NIK number format",
}
400 - Bad Request
Request with invalid date of birth format
Response
1
2
3
4
{
"verification_status": false,
"reason": "Invalid date_of_birth format"
}
503 - Service Unavailable
Dukcapil Server Error
Response
1
2
3
4
5
6
7
8
9
{
"verification_status": false,
"reason": "Something wrong in Dukcapil server",
"result": {
"nik": null,
"name": null,
"date_of_birth": null
}
}