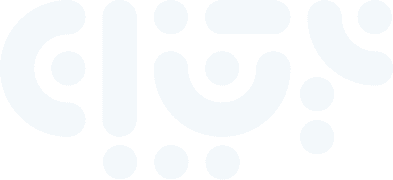
GLAIR Vision SDK
Our SDK lets you make server-side HTTP requests from your server to GLAIR Vision API endpoints. It contains boilerplate code so you can get up and running in just a few lines of code..
The software development kit (SDK) is currently undergoing active development, and as a result, certain APIs may not be readily available directly within the SDK and must be requested directly through the respective endpoints.
Official libraries
Java
Java is a widely-used programming language known for its versatility and robustness. Java is known for its "write once, run anywhere" principle
Go
Go is an open source programming language that makes it simple to build secure, scalable systems
Android
Android is Google's mobile operating system for their line of smartphones and tablets.
NodeJS SDK
Requires Node.js v18 or higher. More details on NPM page.
Installation
1
npm install @glair/vision
Usage
1
2
3
4
5
6
7
8
9
import { Vision } from '@glair/vision';
const vision = new Vision({
apiKey: 'api-key',
username: 'username',
password: 'password',
});
await vision.ocr.ktp({ image: '/path/to/image/KTP.jpg' });
Usage (override Base URL)
1
2
3
4
5
6
7
8
9
10
11
12
import { Vision } from '@glair/vision';
// You can override baseUrl by passing "baseUrl" param. Default value is https://api.vision.glair.ai.
// This is useful if you want to use our staging environment.
const vision = new Vision({
baseUrl: 'https://api.vision.glair.ai',
apiKey: 'api-key',
username: 'username',
password: 'password',
});
await vision.ocr.ktp({ image: '/path/to/image/KTP.jpg' });
Java SDK
Requires Java 8 or higher. More details on Maven Page.
Requirement
- Requires Java 8 or higher.
- Valid GLAIR credentials, including an API key, username, and password.
Integration
Installation (build.gradle)
1
implementation 'ai.glair:glair-vision:0.0.1-beta.1'
Configuration
Option | Default | Description |
---|---|---|
apiKey | default-api-key | Your API Key |
username | default-username | Your username |
password | default-password | Your password |
baseUrl | https://api.vision.glair.ai | Base URL for the API |
apiVersion | v1 | GLAIR Vision API version to be used |
Utilizing SDK
Usage
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
import glair.vision.Vision;
import glair.vision.model.VisionSettings;
public class App {
public static void main(String[] args) {
VisionSettings settings = new VisionSettings.Builder()
.username("username")
.password("password")
.apiKey("api-key")
.build();
Vision vision = new Vision(settings);
String imagePath = "/path/to/image.jpg";
String response = "";
try {
KtpParam param = new KtpParam(imagePath);
response = vision
.ocr()
.ktp(param);
} catch (Exception e) {
response = e.getMessage();
}
System.out.println("Response: " + response);
}
}
Go SDK
Detailed information abou GLAIR Vision Go SDK is available on the repository page.
Requirement
- Go 1.18 or higher with Go Modules.
- Valid GLAIR credentials, including an API key, username, and password.
Integration
Install the SDK to your Go project by executing the following command:
Installation
1
go get -u github.com/glair-ai/glair-vision-go
or, you can directly import the required modules and let the Go toolchain to automatically resolve the dependencies
1
2
3
4
import (
"github.com/glair-ai/glair-vision-go"
"github.com/glair-ai/glair-vision-go/client"
)
Usage
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
package main
import (
"context"
"encoding/json"
"fmt"
"log"
"os"
"github.com/glair-ai/glair-vision-go"
"github.com/glair-ai/glair-vision-go/client"
)
func main() {
ctx := context.Background()
config := glair.NewConfig("", "", "")
client := client.New(config)
file, _ := os.Open("path/to/image.jpg")
result, err := client.Ocr.KTP(ctx, glair.OCRInput{
Image: file,
})
if err != nil {
log.Fatalln(err.Error())
}
jsonResponse, _ := json.MarshalIndent(result, "", " ")
fmt.Println(string(jsonResponse))
}
Configuration
Option | Default | Description |
---|---|---|
BaseUrl | https://api.vision.glair.ai | Base URL for GLAIR Vision API |
ApiVersion | v1 | GLAIR Vision API version to be used |
Client | Default Go HTTP client | HTTP Client to be used when sending request to GLAIR Vision API |
Logger | LeveledLogger with LevelNone | Logger instace to be used to log errors, information, or debugging messages |
Android SDK
This SDK is currently in beta and subject to significant changes in the future. If you wish to use this SDK, please contact us via email at hi[at]glair.ai or through your company's representative.
Requirement
- Android 7 (API Level 24) or above
- Valid GLAIR credentials, including an API key, username, and password.
- The
VisionSDK.zip
file provided by GLAIR.
Integration
- Extract
VisionSDK.zip
to~/.m2/repository.
- Add the following line to the
settings.gradle.kts
file:
1
2
3
4
5
6
7
dependencyResolutionManagement {
repositories {
mavenLocal()
...
}
...
}
- Add the following line to the
build.gradle.kts
file:
1
2
3
4
dependencies {
implementation("ai.glair:vision-android:0.0.1-beta.1")
...
}
Configuration
Here are two configuration classes for Vision SDK:
VisionConfiguration
: for essential and common configuration.<Type>Customization
: for defining specific customizations for each type of SDK.
Begin by configuring the SDK with the following parameters.
Only apiKey
, username
, and password
are required in the VisionConfiguration
class. The rest is optional.
1
2
3
4
5
6
7
import ai.glair.vision.model.VisionConfiguration
val config = VisionConfiguration.builder<KtpCustomization>()
.apiKey("apiKey")
.username("username")
.password("password")
.build()
VisionConfiguration Class
Option | Default | Description |
---|---|---|
apiKey | default-api-key | Your API Key |
username | default-username | Your username |
password | default-password | Your password |
baseUrl | https://api.vision.glair.ai | Base URL for the API |
apiVersion | v1 | GLAIR Vision API version to be used |
logLevel | LogLevel.INFO | Specifies the level of logging output for the logger |
language | Language.EN | Support English (EN) and Bahasa Indonesia (ID). Values stored in Language enum class |
customization | Default | Defaults tailored to each type of SDK customization |
Utilizing KTP Activity
If you prefer not to create your own UI for capturing and reading KTP, use the following code:
Call Activity
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
class MainActivity: AppCompatActivity() {
private var activityResultLauncher =
registerForActivityResult(ActivityResultContracts.StartActivityForResult()) { result ->
processResult(result)
}
private fun initializeSdk() {
val customization = KtpCustomization.Builder()
.backgroundColor("#222222")
.captureButtonColor("#ff0000")
.build()
val config = VisionConfiguration.builder<KtpCustomization>()
.apiKey(apiKey)
.username(username)
.password(password)
.customization(customization)
.build()
val intent = Intent(this@MainActivity, KtpActivity::class.java).apply {
putExtra(KtpActivity.CONFIGURATION, config)
}
activityResultLauncher.launch(intent)
}
private fun processResult(result: ActivityResult) {
if (result.resultCode == KtpActivity.RESULT_CODE && result.data != null) {
val resultData = result.data!!.getStringExtra(KtpActivity.RESULT_KEY)
Log.i("Main App", "Main app here: $resultData")
}
}
}
Call Fragment
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
class MainActivity : AppCompatActivity() {
private lateinit var fragment: KtpFragment
private lateinit var config: VisionConfiguration<KtpCustomization>
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val binding = ActivityTemplateBinding.inflate(layoutInflater)
setContentView(binding.root)
val customization = KtpCustomization.Builder()
.backgroundColor("#222222")
.captureButtonColor("#ff0000")
.build()
val config = VisionConfiguration.builder<KtpCustomization>()
.apiKey("apiKey")
.username("username")
.password("password")
.customization(customization)
.build()
addFragment()
}
private fun addFragment() {
fragment = KtpFragment(config) { result ->
Log.i("Main App", "Main app here: $result")
}
val transaction = supportFragmentManager.beginTransaction()
transaction.replace(R.id.fragment_container, fragment, KtpFragment.TAG)
transaction.commit()
}
}
KtpCustomization Class
Option | Default | Description |
---|---|---|
backgroundColor | #1C1B1F | Background color in Hex String RGB format |
fontColor | #E6E1E5 | Font color in Hex String RGB format |
fontSize | 1f | Font size relative to current GLAIR size |
backColor | #E6E1E5 | Back color in Hex String RGB format |
captureButtonColor | #E6E1E5 | Capture button color in Hex String RGB format |
imagePickerColor | #E6E1E5 | Image Picker color in Hex String RGB format |
flipCameraColor | #E6E1E5 | Flip Camera color in Hex String RGB format |
retryColor | #1C1B1F | Retry color in Hex String RGB format |
checklistColor | #10B982 | Checklist color in Hex String RGB format |
Utilizing Liveness Activity
If you'd rather not create your own UI for Liveness Verification, use the following code:
Call Activity
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
class MainActivity: AppCompatActivity() {
private var activityResultLauncher =
registerForActivityResult(ActivityResultContracts.StartActivityForResult()) { result ->
processResult(result)
}
private fun initializeSdk() {
val customization = LivenessCustomization.Builder()
.backgroundColor("#222222")
.livenessCountdown(5)
.build()
val config = VisionConfiguration.builder<LivenessCustomization>()
.apiKey(apiKey)
.username(username)
.password(password)
.customization(customization)
.build()
val intent = Intent(this@MainActivity, LivenessActivity::class.java).apply {
putExtra(LivenessActivity.CONFIGURATION, config)
}
activityResultLauncher.launch(intent)
}
private fun processResult(result: ActivityResult) {
if (result.resultCode == LivenessActivity.RESULT_CODE && result.data != null) {
val resultData = result.data!!.getStringExtra(LivenessActivity.RESULT_KEY)
Log.i("Main App", "Main app here: $resultData")
}
}
}
Call Fragment
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
class MainActivity : AppCompatActivity() {
private lateinit var fragment: LivenessFragment
private lateinit var config: VisionConfiguration<LivenessCustomization>
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val binding = ActivityTemplateBinding.inflate(layoutInflater)
setContentView(binding.root)
val customization = LivenessCustomization.Builder()
.backgroundColor("#222222")
.livenessCountdown(5)
.build()
val config = VisionConfiguration.builder<LivenessCustomization>()
.apiKey("apiKey")
.username("username")
.password("password")
.customization(customization)
.build()
addFragment()
}
private fun addFragment() {
fragment = LivenessFragment(config) { result ->
Log.i("Main App", "Main app here: $result")
}
val transaction = supportFragmentManager.beginTransaction()
transaction.replace(R.id.fragment_container, fragment, LivenessFragment.TAG)
transaction.commit()
}
}
LivenessCustomization Class
Option | Default | Type | Description |
---|---|---|---|
backgroundColor | #1C1B1F | String | Background Color |
fontColor | #E6E1E5 | String | Foreground Color |
fontSize | 1f | Float | Relative Multiplier for Font Size Based on Current Size |
backColor | #E6E1E5 | String | Button Back Color |
livenessNumberGesture | 1 | Int | Number of Gestures Needed |
progressBarColor | #E6E1E5 | String | Progress Bar Color |
gestureIllustrationColor | #E6E1E5 | String | Gesture Illustration Color Below Camera View |
livenessCountdown | 5 | Int | Total Countdown Time |
Leveraging the Vision API Wrapper
If you prefer to build your own custom UI for capturing and processing Vision API data, you can use our Java API Wrapper.
iOS SDK
This SDK is currently in beta and subject to significant changes in the future. If you wish to use this SDK, please contact us via email at hi[at]glair.ai or through your company's representative.
Requirement
- iOS 13 or higher.
- The
VisionSDK.xcframework
framework provided by GLAIR. - Valid GLAIR credentials, including an API key, username, and password.
Integration
- Drag and drop the VisionSDK.xcframework folder into the Frameworks group in your Xcode project's navigator.
- Make sure the VisionSDK.xcframework is set to "Embed & Sign" in the Frameworks, Libraries, and Embedded Content section of your app's target settings.
Configuration
Begin by configuring the SDK with the following params. Only apiKey
, username
, and password
are required. The rest is optional.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
import VisionApp
let config = VisionConfig(
apiKey: "apiKey",
username: "username",
password: "password",
baseUrl: "https://your-be-url",
apiVersion: "v1",
livenessNumberGesture: 2,
livenessCountdown: 3,
language: "id",
backgroundColor: "FFFFFF",
fontColor: "000000",
fontSize: 16,
captureButtonColor: "FF0000"
)
VisionApp.sharedInstance.configure(config)
Option | Default | Description |
---|---|---|
apiKey | default-api-key | Your API Key |
username | default-username | Your username |
password | default-password | Your password |
baseUrl | https://api.vision.glair.ai | Base URL for the API |
apiVersion | v1 | GLAIR Vision API version to be used |
livenessNumberGesture | 3 | Number of gesture in Liveness SDK (Min: 1, Max: 3) |
livenessCountdown | 3 | Countdown in Liveness SDK (Min: 3, Max: 5) |
language | en | Support English (en) and Bahasa Indonesia (id) |
backgroundColor | #000000 | Background color in RGB or RGBA format |
fontColor | #FFFFFF | Font color in RGB or RGBA format |
fontSize | 17 | Font size |
captureButtonColor | #FFFFFF | Capture button color in RGB or RGBA format |
Utilizing KTP View Controller
If you prefer not to create your own UI for capturing and reading KTP, use the following code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
// Set close app callback for navigation
VisionApp.sharedInstance.setCloseAppCallback {
presentationMode.wrappedValue.dismiss()
}
// Utilize the GLAIR Vision KTP UIViewController:
let viewController = VisionApp.sharedInstance.ktpView({ result in
// get result properties: ktp result, captured image, and error
})
// Utilize the GLAIR Vision Liveness UIViewController:
let viewController = VisionApp.sharedInstance.livenessView({ result in
// get result properties: success and response histories
})
Leveraging the OCR KTP API Wrapper
If you'd rather create your own UI from scratch for capturing and reading KTP, you can use our API wrapper:
1
2
3
4
5
6
// OCR KTP
let ktp = try await VisionApp.sharedInstance.ktp(imageData)
// Liveness
let activeLiveness = try await VisionApp.sharedInstance.activeLiveness(imageData, gestureCode)
let passiveLiveness = try await VisionApp.sharedInstance.passiveLiveness(imageData)