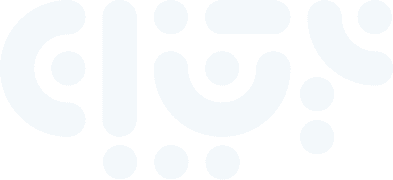
Passive Liveness
Passive liveness detection occurs in the background to detect liveness, hence the “passive” descriptor, and does not require the user to perform actions such as blinking, smiling, or moving their head.
Passive Liveness Object
- Name
status
- Type
- string
- Description
A short string indicating the status of the result.
success
failed
- Name
result
- Type
- object
- Description
The result of passive liveness.
- Name
spoof_percentage
- Type
- number
- Description
How much the faces match each other (in percentage).
- Name
status
- Type
- string
- Description
Enum code indicating the detection result. See Responses below for details.
HIGH
MODERATE
LOW
face not detected
too many faces detected
Passive Liveness
Detect someone's passive liveness.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg)
- Description
The image file to be detected.
Sample Request
1
2
3
4
5
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.faceBio.passiveLiveness({ image: '/path/to/image/Face.jpg' });
Sample Response
1
2
3
4
5
6
7
{
"status": "success",
"result": {
"spoof_percentage": 0.02611,
"status": "LOW"
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Success, high spoof percentage.
// TODO: status should be "failed" due to high spoof percentage?
Response
1
2
3
4
5
6
7
{
"status": "success",
"result": {
"spoof_percentage": 0.99768,
"status": "HIGH"
}
}
200 - OK
Success, moderate spoof percentage.
Response
1
2
3
4
5
6
7
{
"status": "success",
"result": {
"spoof_percentage": 0.5678,
"status": "MODERATE"
}
}
200 - OK
Success, low spoof percentage.
Response
1
2
3
4
5
6
7
{
"status": "success",
"result": {
"spoof_percentage": 0.02611,
"status": "LOW"
}
}
200 - OK
Failed, no face detected.
Response
1
2
3
4
5
6
7
{
"status": "failed",
"result": {
"spoof_percentage": 1.0,
"status": "face not detected"
}
}
200 - OK
Failed, too many faces.
Response
1
2
3
4
5
6
7
{
"status": "failed",
"result": {
"spoof_percentage": 1.0,
"status": "too many faces detected"
}
}
400 - Bad Request
Invalid file format for captured_image
.
// TODO: no captured image
Response
1
2
3
4
{
"status": "failed",
"error_message": "enter valid base64 for captured image",
}
400 - Bad Request
Invalid file format for stored_image
.
// TODO: no captured image
Response
1
2
3
4
{
"status": "failed",
"error_message": "enter valid format image for captured image",
}
500 - Internal Server Error
GLAIR Vision API server error.
Response
1
2
3
4
{
"status": "failed",
"error_message": "internal server error"
}