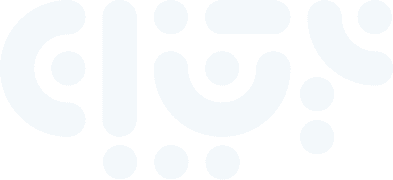
Paspor (Passport)
Paspor (Passport).
Paspor Object
- Name
status
- Type
- string
- Description
Enum code indicating the status of the reading result.
SUCCESS
NO_FILE
FILE_INVALID_FORMAT
FAILED
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
read
- Type
- object
- Description
Contains the reading for each Passport fields. Each fields has
confidence
(in percentage) andvalue
(the reading).- Name
birth_date
- Type
- object
- Description
Date of birth in dd-mm-yyyy format.
- Name
birth_date_hash
- Type
- object
- Description
Date of birth hash.
- Name
country
- Type
- object
- Description
Country.
- Name
doc_number
- Type
- object
- Description
Document number.
- Name
doc_number_hash
- Type
- object
- Description
Document number hash.
- Name
document_type
- Type
- object
- Description
Document type.
- Name
expiry_date
- Type
- object
- Description
Expiry date in dd-mm-yyyy format.
- Name
expiry_date_hash
- Type
- object
- Description
Expiry date hash.
- Name
final_hash
- Type
- object
- Description
Final hash.
- Name
name
- Type
- object
- Description
Name.
- Name
nationality
- Type
- object
- Description
Nationality.
- Name
optional_data
- Type
- object
- Description
Optional data.
- Name
optional_data_hash
- Type
- object
- Description
Optional data hash.
- Name
sex
- Type
- object
- Description
Sex.
- Name
surname
- Type
- object
- Description
Surname.
Read Passport
Detects a valid Passport image and returns the information that contain on MRZ code (located at the bottom of the passport).
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg)
- Description
The image file for the Passport.
Sample Request
1
2
3
4
5
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.ocr.passport({ image: '/path/to/image/Passport.jpg' });
Sample Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
{
"status": "SUCCESS",
"reason": "File successfully read.",
"read": {
"birth_date": {
"confidence": 99,
"value": "01-02-1987"
},
"birth_date_hash": {
"confidence": 99,
"value": "1"
},
"country": {
"confidence": 99,
"value": "IDN"
},
"doc_number": {
"confidence": 99,
"value": "ABC01234"
},
"doc_number_hash": {
"confidence": 99,
"value": "1"
},
"document_type": {
"confidence": 99,
"value": "P"
},
"expiry_date": {
"confidence": 99,
"value": "06-07-2008"
},
"expiry_date_hash": {
"confidence": 99,
"value": "7"
},
"final_hash": {
"confidence": 99,
"value": "3"
},
"name": {
"confidence": 99,
"value": "NAMA"
},
"nationality": {
"confidence": 99,
"value": "IDN"
},
"optional_data": {
"confidence": 99,
"value": "01234567890"
},
"optional_data_hash": {
"confidence": 99,
"value": "8"
},
"sex": {
"confidence": 99,
"value": "M"
},
"surname": {
"confidence": 99,
"value": "PANGGILAN"
}
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with readable Passport image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File successfully read.",
//...,
}
200 - OK
Request with non Passport image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File successfully read. Some fields are invalid.",
//...,
}
400 - Bad Request
Request without form-data image
Response
1
2
3
4
5
{
"status": "NO_FILE",
"reason": "No file in request body",
//...,
}
415 - Unsupported Media Type
Request with non-image file format
Response
1
2
3
4
5
{
"status": "FILE_INVALID_FORMAT",
"reason": "Failed to process invalid file format. Please upload the correct file format",
//...,
}