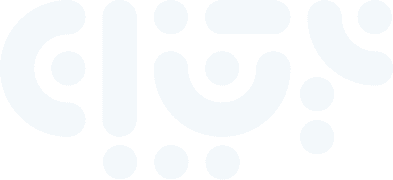
Kartu Tanda Penduduk (KTP)
KTP is an identity card issued in Indonesia.
KTP Object
- Name
status
- Type
- string
- Description
Enum code indicating the status of the reading result.
SUCCESS
NO_FILE
FILE_INVALID_FORMAT
FAILED
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
qualities
- Type
- object
- Description
When the reading is successful, you don't need to care about
qualities
. When our engine thinks that there's a problem in the uploaded image, thisqualities
object will tell you what's wrong.- Name
is_blurred
- Type
- boolean
- Description
If
true
, the image uploaded is too blurry. Try re-uploading with a less blurry image.
- Name
is_bright
- Type
- boolean
- Description
If
true
, the image uploaded is too bright. Try re-uploading with normal lighting.
- Name
is_copy
- Type
- boolean
- Description
If
true
, the image uploaded is from a photocopier. Try re-uploading the real one.
- Name
is_cropped
- Type
- boolean
- Description
If
true
, the image uploaded is cropped. Try re-uploading the whole image.
- Name
is_dark
- Type
- boolean
- Description
If
true
, the image uploaded is too dark. Try re-uploading with normal lighting.
- Name
is_flash
- Type
- boolean
- Description
If
true
, the image uploaded contains flash obstructing parts of the image. Try re-uploading with normal lighting.
- Name
is_ktp
- Type
- boolean
- Description
If
true
, the image uploaded is considered a KTP. That's good.
- Name
is_rotated
- Type
- boolean
- Description
If
true
, the image uploaded is rotated. Try re-uploading with portrait orientation.
- Name
is_taken_from_screen
- Type
- boolean
- Description
If
true
, the image uploaded is captured / taken from screen. Try re-uploading with image that captured real ktp.
- Name
images
- Type
- object
- Description
Contains the cropped image (in
base64
format) of KTP's face and signature.- Name
photo
- Type
- string
- Description
Face in
base64
format.
- Name
sign
- Type
- string
- Description
Signature in
base64
format.
- Name
read
- Type
- object
- Description
Contains the reading for each KTP fields. Each fields has
confidence
(in percentage) andvalue
(the reading).- Name
agama
- Type
- object
- Description
Religion
- Name
alamat
- Type
- object
- Description
Address.
- Name
berlakuHingga
- Type
- object
- Description
Expiration date.
- Name
golonganDarah
- Type
- object
- Description
Blood type.
- Name
jenisKelamin
- Type
- object
- Description
Sex.
- Name
kecamatan
- Type
- object
- Description
Prefecture.
- Name
kelurahanDesa
- Type
- object
- Description
District.
- Name
kewarganegaraan
- Type
- object
- Description
Nationality.
- Name
kotaKabupaten
- Type
- object
- Description
City.
- Name
nama
- Type
- object
- Description
Name.
- Name
nik
- Type
- object
- Description
ID number.
- Name
pekerjaan
- Type
- object
- Description
Job.
- Name
provinsi
- Type
- object
- Description
Province.
- Name
rtRw
- Type
- object
- Description
Neighborhood number.
- Name
statusPerkawinan
- Type
- object
- Description
Marital status.
- Name
tanggalLahir
- Type
- object
- Description
Date of birth.
- Name
tempatLahir
- Type
- object
- Description
Place of birth.
Read KTP
Detects a valid KTP image and returns the information as text.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg, .pdf, .tiff)
- Description
The image file for the KTP.
Sample Request
1
2
3
4
5
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.ocr.ktp({ image: '/path/to/image/KTP.jpg' });
Sample Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
{
"status": "SUCCESS",
"reason": "File Successfully Read",
"images": {
"photo": "/9j/4AAQSkZJRgABAQAAAQABAAD/...",
"sign": "/9j/4AAQSkZJRgABAQAAAQABAAD/..."
},
"read": {
"agama": {
"confidence": 98,
"value": "ISLAM"
},
"alamat": {
"confidence": 87,
"value": "JUS AGUNG WAY HALIM"
},
"berlakuHingga": {
"confidence": 97,
"value": "SEUMUR HIDUP"
},
"golonganDarah": {
"confidence": 90,
"value": "-"
},
"jenisKelamin": {
"confidence": 93,
"value": "LAKI-LAKI"
},
"kecamatan": {
"confidence": 99,
"value": "KEDATON"
},
"kelurahanDesa": {
"confidence": 99,
"value": "KEDATON"
},
"kewarganegaraan": {
"confidence": 99,
"value": "WNI"
},
"kotaKabupaten": {
"confidence": 97,
"value": "BANDAR LAMPUNG"
},
"nama": {
"confidence": 97,
"value": "NICOJULIAN"
},
"nik": {
"confidence": 99,
"value": "1871010907930009"
},
"pekerjaan": {
"confidence": 95,
"value": "PELAJAR / MAHASISWA"
},
"provinsi": {
"confidence": 99,
"value": "LAMPUNG"
},
"rtRw": {
"confidence": 88,
"value": "014/000"
},
"statusPerkawinan": {
"confidence": 98,
"value": "BELUM KAWIN"
},
"tanggalLahir": {
"confidence": 97,
"value": "09-07-1993"
},
"tempatLahir": {
"confidence": 97,
"value": "BANDAR LAMPUNG"
}
}
}
Read KTP with Qualities
Returns the qualities of KTP image and the KTP information as text.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg, .pdf, .tiff)
- Description
The image file for the KTP.
Sample Request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
// Minimum Node 18. Save the code as 'index.mjs' and run it by executing 'node index.mjs'
import { readFileSync } from "fs";
const url = 'https://api.vision.glair.ai/ocr/v1/ktp/qualities';
const basicAuth = 'Basic ' + Buffer.from('USERNAME' + ':' + 'PASSWORD').toString('base64');
const apiKey = 'API_KEY';
const formData = new FormData();
formData.append('image', new Blob([readFileSync('/path/to/image/KTP.jpeg')]));
const config = {
method: 'POST',
headers: {
Authorization: basicAuth,
'x-api-key': apiKey,
},
body: formData,
};
const response = await fetch(url, config);
console.log(await response.json());
Sample Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
{
"status": "SUCCESS",
"reason": "File Successfully Read",
"qualities": {
"is_blurred": false,
"is_bright": false,
"is_copy": false,
"is_cropped": false,
"is_dark": false,
"is_flash": false,
"is_ktp": true,
"is_rotated": false,
"is_taken_from_screen": false
},
"images": {
"photo": "/9j/4AAQSkZJRgABAQAAAQABAAD/...",
"sign": "/9j/4AAQSkZJRgABAQAAAQABAAD/..."
},
"read": {
// KTP fields
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with readable KTP image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File Successfully Read",
//...,
}
200 - OK
Request with non KTP File
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File successfully read. Some fields are invalid.",
//...,
}
200 - Unrecognized Document Type
(With Qualities Only) Request with unrecognized document type
Response
1
2
3
4
5
{
"status": "UNRECOGNIZED_DOCUMENT_TYPE",
"reason": "Unrecognized document type detected.",
//...,
}
200 - Multiple Documents
(With Qualities Only) Request with multiple documents
Response
1
2
3
4
5
{
"status": "MULTIPLE_DOCUMENTS_DETECTED",
"reason": "Multiple documents detected.",
//...,
}
400 - Bad Request
Request without form-data image
Response
1
2
3
4
5
{
"status": "NO_FILE",
"reason": "No file in request body",
//...,
}
415 - Unsupported Media Type
Request with non-image file format
Response
1
2
3
4
5
{
"status": "FILE_INVALID_FORMAT",
"reason": "Failed to process invalid request",
//...,
}