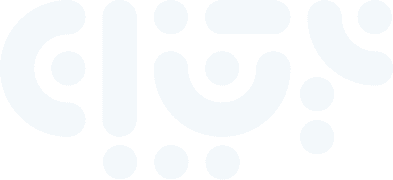
General Document (General Document)
General Document refers to any type of document that can be processed using the OCR (Optical Character Recognition) API. It encompasses a wide range of document types, including but not limited to handwriting samples, receipts, invoices, and various supported file formats.
General Document Object
- Name
status
- Type
- string
- Description
Enum code indicating the status of the reading result.
SUCCESS
NO_FILE
FILE_INVALID_FORMAT
FAILED
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
read
- Type
- object
- Description
Contains the reading for General Document fields.
- Name
all_texts
- Type
- array of object
- Description
Array of all texts available in image. Each element has
polygon
(coordinates of the value: top-left, top-right, bottom-right, bottom-left) andvalue
(the reading).
- Name
tables
- Type
- array of object
- Description
Array of all tables available in the image.
Read General Document
Detects a valid General Document image and returns the information as text.
Required parameter
- Name
image
- Type
- file (.png, .jpg, .jpeg, .tiff, .pdf)
- Description
The image file for the General Document.
Optional parameter
- Name
table
- Type
- boolean
- Description
(By default) if not set or set to false, the OCR will not return table data. If set to true, it will return OCR results for tables.
Sample Request
1
2
3
4
5
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.ocr.generalDocument({ image: '/path/to/image/General-Document.jpg' });
Sample Response
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
{
"status": "SUCCESS",
"reason": "File Successfully Read",
"read": {
"all_texts": [
{
"value": "THE QUICK BROWN FOX",
"page_index": 0,
"polygon": [[209, 17],[640, 14],[640, 44],[209, 47]],
"confidence": 95,
"confidence_text": 95
},
{
"value": "JUMPS OVER THE LAZY FOG",
"page_index": 0,
"polygon": [[241, 63],[602, 62],[602, 84],[242, 86]],
"confidence": 95,
"confidence_text": 95
},
...
],
"tables": [
{
"page_index": 0,
"row_count": 8,
"column_count": 5,
"polygon": [[60, 204], [454, 204], [453, 370], [60, 370]]
"cells": [
{
"row_index": 0,
"column_index": 0,
"row_span": 2,
"column_span": 1,
"is_header": false,
"is_projected_row_header": false,
"value": "ABC",
"polygon": [[68, 246], [111, 246], [111, 276], [68, 276]],
"confidence": 97.6,
"confidence_text": 97.6
},
{
"row_index": 1,
"column_index": 1,
"row_span": 1,
"column_span": 1,
"is_header": false,
"is_projected_row_header": false,
"value": "CDE",
"polygon": [[198, 276], [242, 276], [242, 307], [198, 307]],
"confidence": 97.6,
"confidence_text": 97.6
}
]
},
...
]
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint, in addition to general responses specified in Errors.
200 - OK
Request with readable General Document image
Response
1
2
3
4
5
{
"status": "SUCCESS",
"reason": "File Successfully Read",
//...,
}
// TODO: no "Request with non General Document File"?
400 - Bad Request
Request without form-data image
Response
1
2
3
4
5
{
"status": "NO_FILE",
"reason": "No file in request body",
//...,
}
415 - Unsupported Media Type
Request with non-image file format
Response
1
2
3
4
5
{
"status": "FILE_INVALID_FORMAT",
"reason": "Failed to process invalid file format. Please upload the correct file format",
//...,
}