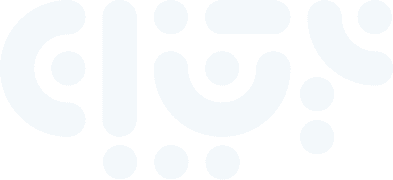
Face Verification (KTP)
Verify some KTP field and owner face by NIK to Dukcapil database.
Face Verification Object
- Name
verification_status
- Type
- boolean
- Description
If
true
, the uploaded KTP data is valid and correct.
- Name
reason
- Type
- string
- Description
A human-readable message providing more details about the reading result.
- Name
data
- Type
- object
- Description
Contains the verification status for each inputted KTP fields.
- Name
nik
- Type
- boolean | null
- Description
If
true
, the uploaded NIK is valid and exists in Dukcapil database.
- Name
name
- Type
- boolean | null
- Description
If
true
, the uploaded name field is valid and corresponds with given NIK.
- Name
date_of_birth
- Type
- boolean | null
- Description
If
true
, the uploaded date of birth field is valid and corresponds with given NIK.
- Name
face_image_percentage
- Type
- 1-100 | null
- Description
Face match percentage from Dukcapil.
Face Verification
Verify some KTP field and owner face by NIK to Dukcapil database and return the verification result.
Required parameter
- Name
nik
- Type
- string
- Description
The
NIK
field in KTP.
- Name
name
- Type
- string
- Description
The
Nama
field in KTP.
- Name
date_of_birth
- Type
- string
- Description
The
Tanggal Lahir
field in KTP withdd-mm-yyyy
format.
- Name
face_image
- Type
- file (.png, .jpg, .jpeg)
- Description
The face image of KTP owner.
Sample Request
1
2
3
4
5
6
7
8
9
10
import { Vision } from '@glair/vision';
const vision = new Vision({ apiKey: 'api-key', username: 'username', password: 'password' });
await vision.identity.faceVerification({
nik: 'NIK';,
name: 'Nama',
date_of_birth: '01-02-1999', // dd-mm-yyyy
face_image: '/path/to/image/face.jpg',
});
Sample Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": true,
"reason": "Verification Success",
"result": {
"nik": true,
"name": true,
"date_of_birth": true,
"face_image_percentage": 82.04
}
}
Request ID
An associated request identifier is generated for every request made to this endpoint.
This value can be found in the response headers under Request-Id
Responses
Various responses for this endpoint.
200 - OK
Request with valid and verified KTP data and owner face match similarity above 75%
Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": true,
"reason": "Verification Success",
"result": {
"nik": true,
"name": true,
"date_of_birth": true,
"face_image_percentage": 82.04
}
}
200 - OK
Request with a NIK doesn't exist in Dukcapil
Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": false,
"reason": "NIK not found on Dukcapil database",
"result": {
"nik": false,
"name": null,
"date_of_birth": null,
"face_image_percentage": 0
}
}
200 - OK
Request with field name doesn't match with given NIK
Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": false,
"reason": "Name does not match",
"result": {
"nik": true,
"name": false,
"date_of_birth": true,
"face_image_percentage": 82.04
}
}
200 - OK
Request with field date of birth doesn't match with given NIK
Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": false,
"reason": "Date of birth does not match",
"result": {
"nik": true,
"name": true,
"date_of_birth": false,
"face_image_percentage": 82.04
}
}
200 - OK
Request with owner face match similarity under 75%
Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": false,
"reason": "Selfie image does not pass the threshold",
"result": {
"nik": true,
"name": true,
"date_of_birth": true,
"face_image_percentage": 42.04
}
}
400 - Bad Request
Request with invalid NIK number
Response
1
2
3
4
{
"verification_status": false,
"reason": "Invalid NIK number format",
}
400 - Bad Request
Request with invalid date of birth format
Response
1
2
3
4
{
"verification_status": false,
"reason": "Invalid date_of_birth format"
}
503 - Service Unavailable
Dukcapil Server Error
Response
1
2
3
4
5
6
7
8
9
10
{
"verification_status": false,
"reason": "Something wrong in Dukcapil server",
"result": {
"nik": null,
"name": null,
"date_of_birth": null,
"face_image_percentage": null
}
}